Imagine a world where your Rust programs could simultaneously handle multiple tasks, responding lightning-fast to user requests while efficiently managing resources. This isn’t a futuristic fantasy; it’s the power of asynchronous programming, a crucial tool for building modern, responsive software. And if you’re diving into this exciting world, Carl Fredrik Samson’s “Asynchronous Programming in Rust” is your indispensable guide.
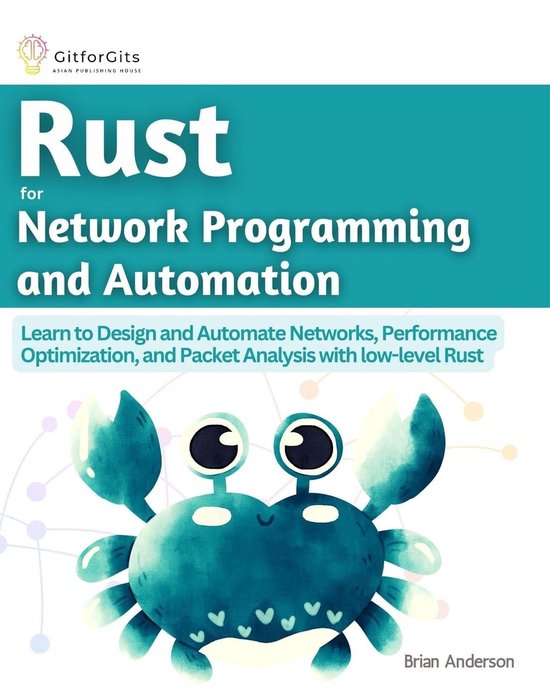
Image: www.bol.com
This book isn’t just for seasoned Rust developers; it’s a welcoming companion for beginners venturing into the realm of concurrency. Samson’s clear, concise explanations and practical examples make even the most complex concepts digestible, leaving you confident in your understanding of asynchronous programming.
Unraveling the Mystery of Async
Asynchronous programming fundamentally changes how your code interacts with the world. While traditional programming involves executing tasks one after another, async allows your code to gracefully handle multiple tasks concurrently, even if those tasks require waiting for external resources like network requests or file operations.
Imagine you’re ordering pizza. In a traditional synchronous program, you’d have to wait for the entire pizza-making process to finish before you could enjoy your meal. But with asynchronous programming, you can initiate the order, go about your day, and receive a notification when your pizza is ready. Your time isn’t wasted waiting; you’re free to do other things while the pizza is being prepared.
The Power of Futures
Central to asynchronous programming in Rust is the concept of futures. A future represents the eventual result of an asynchronous operation, much like a promise of a delicious pizza. It’s a placeholder for the value you’ll eventually receive, allowing your code to keep running while the task is in progress.
Samson’s book carefully introduces futures, demystifying their workings. You’ll learn how to create, manipulate, and combine futures to manage asynchronous operations effectively. The book also covers essential concepts like async blocks, async functions, and the different types of futures, arming you with the tools to write efficient and scalable asynchronous code.
Navigating Through the Complexities of Concurrency
Asynchronous programming opens a world of possibilities, but it also introduces new challenges. Concurrency, the ability to execute multiple tasks simultaneously, requires careful management to prevent race conditions, deadlocks, and other pitfalls. Samson’s guide skillfully addresses these concerns, providing practical strategies and best practices for building robust concurrent systems.
You’ll learn about the various techniques for managing concurrency, including mutexes, channels, and threads. The book also explores real-world scenarios, like parallel processing, message passing, and handling multiple user requests concurrently. With each example, Samson provides valuable insights and code snippets to guide you through the intricacies of asynchronous programming.

Image: www.researchgate.net
Unlocking the Potential of Asynchronous Programming
The payoff for mastering asynchronous programming in Rust is immense. Your programs will become more responsive, scaling effortlessly to handle increasing workloads. You’ll be able to build applications that seamlessly interact with the real world, taking advantage of network resources and user events without sacrificing performance.
Imagine building a high-performance web server that can handle hundreds of simultaneous connections without breaking a sweat, or a game that responds smoothly to player actions despite complex animations and physics simulations. These are just glimpses of the possibilities unlocked by asynchronous programming.
Real-World Applications: The Promise Delivered
Beyond theoretical concepts, Samson’s book demonstrates the practical application of asynchronous programming in real-world scenarios. He delves into topics like network programming, using asynchronous libraries like Tokio to build performant network applications. He also explores the use of asynchronous programming for event-driven systems, making your programs more reactive and efficient.
Here’s a snippet from Carl Fredrik Samson’s book:
- “Asynchronous programming is about writing code that can handle multiple tasks without blocking. This is especially important when dealing with I/O operations, such as reading from a file or making a network request. When you make a network request, your program usually has to wait for the response. This waiting time can be significant if the connection is slow or if the server is busy. With asynchronous programming, your program can start working on other tasks while it’s waiting for the response. When the response arrives, your program can then process it and continue on its way. As a result, asynchronous programming can make your program much more efficient and responsive, especially when dealing with I/O-bound tasks.”*
By the end of this book, you won’t just understand the concepts; you’ll be equipped to build robust, responsive, and scalable applications using the power of asynchronous programming in Rust.
Carl Fredrik Samson Asynchronous Programming In Rust Pdf
Ready to Dive In?
Reading “Asynchronous Programming in Rust” by Carl Fredrik Samson is a journey into the heart of efficient programming in Rust. The book’s content is a testament to the author’s expertise and passion for making asynchronous programming accessible to developers of all levels. Start your journey today and unlock the true potential of your Rust code.